In this article I look at a trigger derived static attribute and demonstrate how to write tests for it.
Test function
For this demo, I have chosen the static attribute BB.TEMPLATE of the standard Asset Control interface for the data vendor Bloomberg. This attribute is trigger derived, i.e. a formula is run every time one of its underlying attributes changes.
You can see this in the AC Desktop:
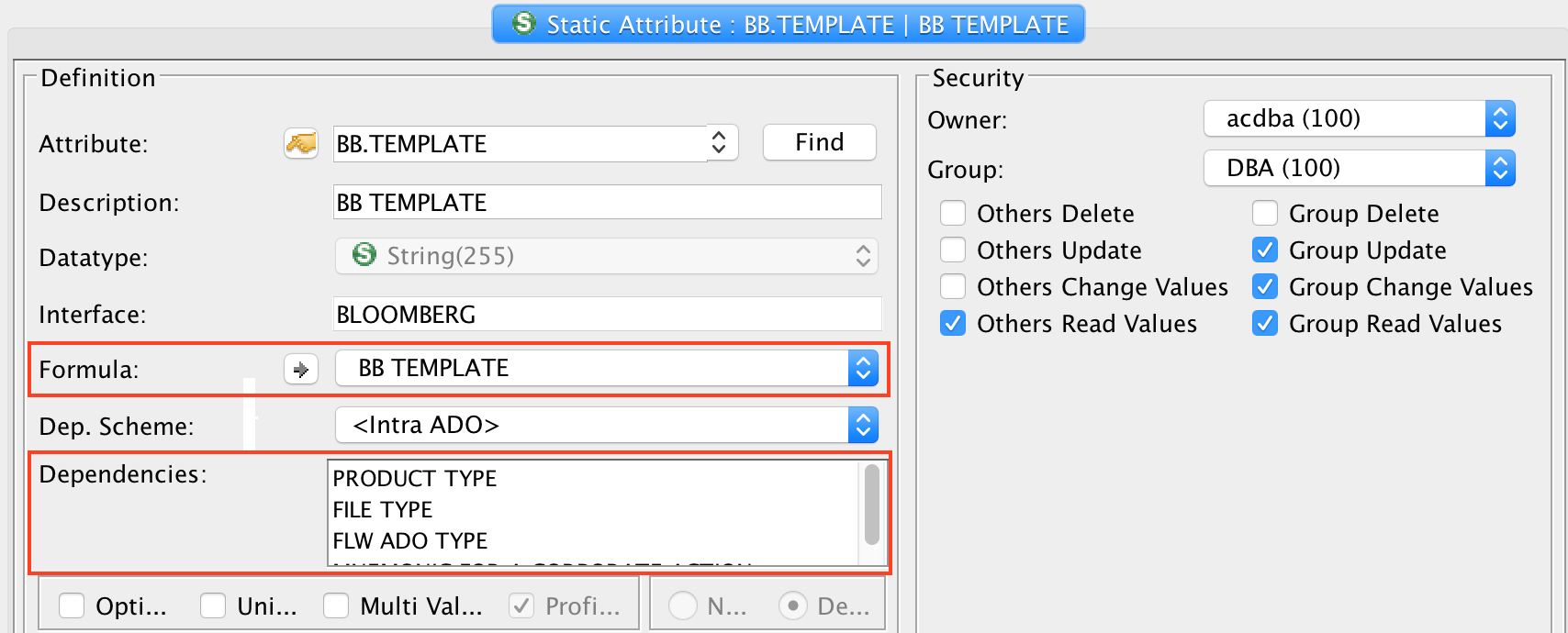
The formula attached to BB.TEMPLATE contains the logic that - dependent on the values of a number of underlying attributes - produces a value for the default template to assign to a BB ADO:
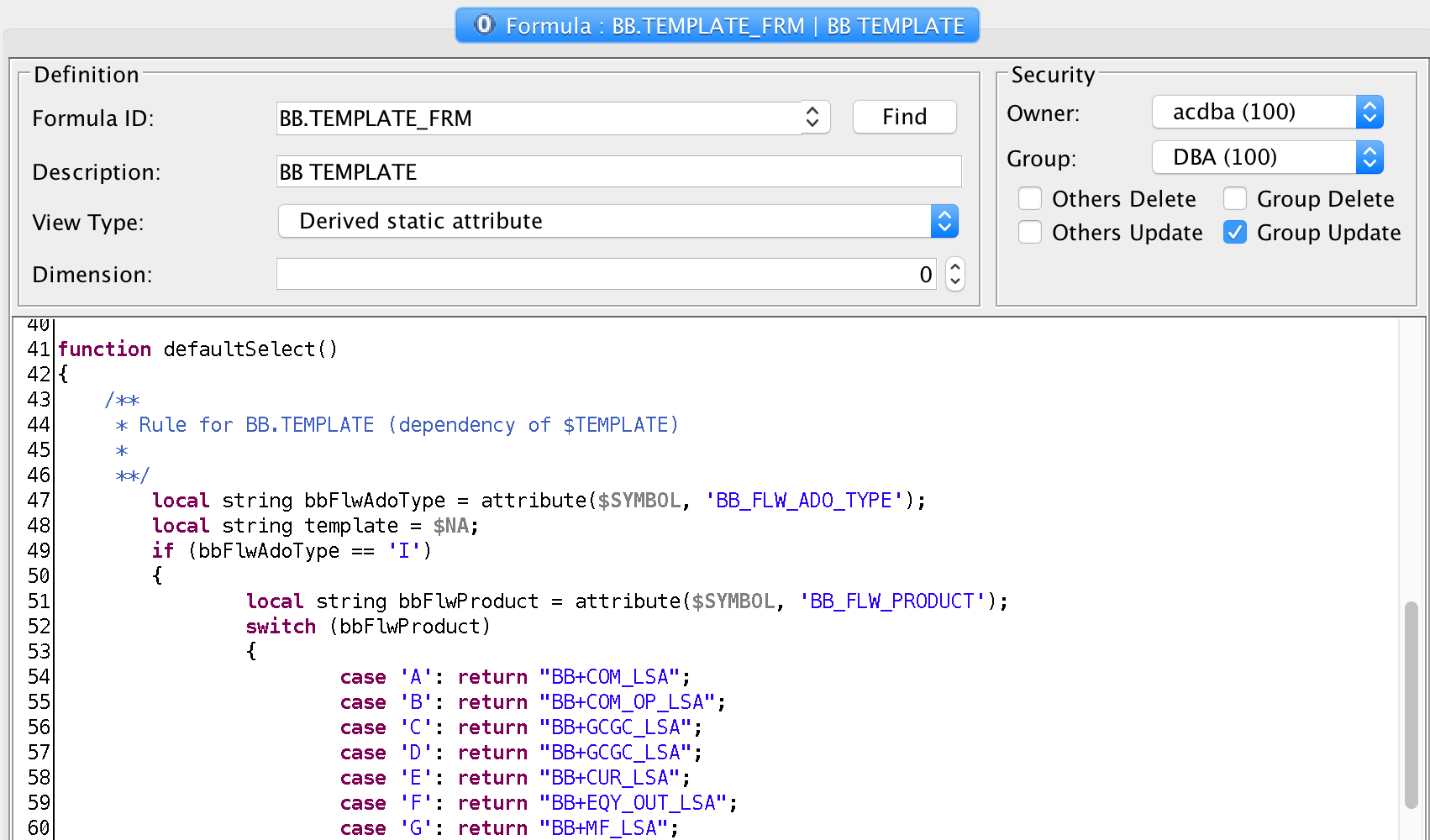
I will now use this in an example of how to write an integration test for a trigger derived static attribute:
Test implementation
As in the previous article, the test is based on the Basic Java API and JUnit 4:
package io.terrafino.ac;
import io.terrafino.api.ac.AcException;
import io.terrafino.api.ac.ado.Ado;
import io.terrafino.api.ac.service.AcConnection;
import io.terrafino.api.ac.service.AcService;
import org.junit.*;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.is;
public class BloombergDefaultTemplateIntegrationTest {
private static AcConnection conn;
private static AcService ac;
private Ado ado;
@BeforeClass
public static void setUp() throws Exception {
conn = AcConnection.getDefaultConnection();
ac = new AcService(conn);
}
@AfterClass
public static void tearDown() throws Exception {
conn.disconnect();
}
@Before
public void setUpTest() throws Exception {
ado = ac.testAdoWithPrefix("BB").withTemplate("BB_LSA").createInAc();
}
@After
public void tearDownTest() throws Exception {
if (ado != null && ado.existsInAc()) {
ado.delete();
}
}
@Test
public void derivesTemplateForAdoTypeIAndProductAOk() throws Exception {
whenWeSetAdoTypeAndProductTo("I", "A");
thenTheTemplateShouldBe("BB+COM_LSA");
}
@Test
public void derivesTemplateForAdoTypeIAndProductBOk() throws Exception {
whenWeSetAdoTypeAndProductTo("I", "B");
thenTheTemplateShouldBe("BB+COM_OP_LSA");
}
// ... more tests ...
private void whenWeSetAdoTypeAndProductTo(String adoType, String product) throws AcException {
ado.set("BB_FLW_ADO_TYPE", adoType);
ado.set("BB_FLW_PRODUCT", product);
ado.store();
}
private void thenTheTemplateShouldBe(String template) throws AcException {
assertThat(ado.load("BB.TEMPLATE").toString(), is(template));
}
}
A few comments on the code above:
- The use of AcConnection, AcService and the two methods (annotated with @BeforeClass and @AfterClass) to set up and tear down the test run as a whole are already familiar.
- New is the Ado instance.
- The method setUpTest annotated with @Before takes care of creating a new test ADO for each test that is run.
- The method AcService.testAdoWithPrefix will create an ADO with an ID like BB.0001.TEST.
- The method tearDownTest annotated with @After will ensure the test ADO is deleted after executing a test.
- Then we see a number of test methods, each annotated with @Test.
- All test methods follow the same pattern:
- Set ADO type and product.
- Ensure that BB.TEMPLATE returns the expected value.
- You can see the corresponding methods whenWeSetAdoTypeAndProductTo and thenTheTemplateShouldBe at the end.
I hope you can see how this pattern can easily be applied and extended for other (trigger) derived attributes.
Thank you for reading!
In the next article I will look at an integration test for Timeseries Validations.